Docs
Components
Avatar
Avatar
An image element with a fallback for representing the user.
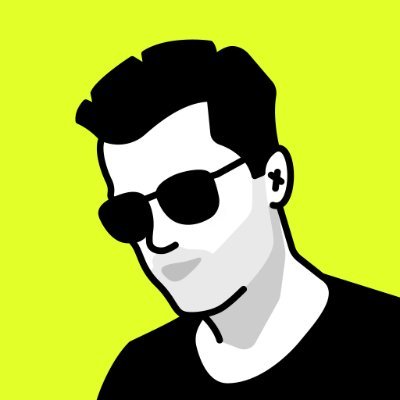
<%= avatar() do %>
<%= avatar_image(src: "https://pbs.twimg.com/profile_images/1806221902727217153/yzQko4Ez_400x400.jpg") %>
<%= avatar_fallback(text: "DS") %>
<% end %>
Installation
1
Copy and paste the following code into your project.
Create a new file in
app/components/ui/avatar_component.rb
and paste the following code:# frozen_string_literal: true
module Ui
# Renders a container for an avatar with customizable styling
class AvatarComponent < ViewComponent::Base
SIZES = {
xs: 'h-6 w-6',
sm: 'h-8 w-8',
md: 'h-10 w-10',
lg: 'h-12 w-12',
xl: 'h-14 w-14'
}
def initialize(size: :md, class_name: nil, **options)
super
@size = size.to_sym
@class_name = class_name
@options = options
end
def call
tag.div(class: avatar_classes, **@options) do
content
end
end
private
def avatar_classes
"relative flex shrink-0 overflow-hidden rounded-full #{size_class} #{@class_name}"
end
def size_class
SIZES[@size] || SIZES[:md]
end
end
# Renders an image for use within an avatar component
class AvatarImageComponent < ViewComponent::Base
def initialize(src:, alt: '', class_name: nil, **options)
super
@src = src
@alt = alt
@class_name = class_name
@options = options
end
def call
tag.img(src: @src, alt: @alt, class: image_classes, **@options)
end
private
def image_classes
"aspect-square h-full w-full #{@class_name}"
end
end
# Renders a fallback element for an avatar when an image is not available
class AvatarFallbackComponent < ViewComponent::Base
def initialize(delay_ms: 600, size: :md, class_name: nil, **options)
super
@delay_ms = delay_ms
@size = size.to_sym
@class_name = class_name
@options = options
end
def call
tag.div(class: fallback_classes, data: { delay_ms: @delay_ms }, **@options) do
content
end
end
private
def fallback_classes
"flex items-center justify-center rounded-full bg-muted #{size_class} #{@class_name}"
end
def size_class
AvatarComponent::SIZES[@size] || AvatarComponent::SIZES[:md]
end
end
end
Coming soon.